Full-Page Takeover (Advanced)
We inject your Question Stream on your order confirmation page via a form embed (not an iframe) allowing for unlimited flexibility and improved security. The below is a code snippet that will make your Question Stream a full page takeover on your order confirmation page. Paste the snippet in your Additional Scripts section of your Checkout settings or in the checkout.liquid
file of your theme.
Your customers are taken to their order confirmation page after they complete their stream of questions. Alternatively, they can click "Go To Order Details" to skip the question. We recommend working with a developer to implement this.
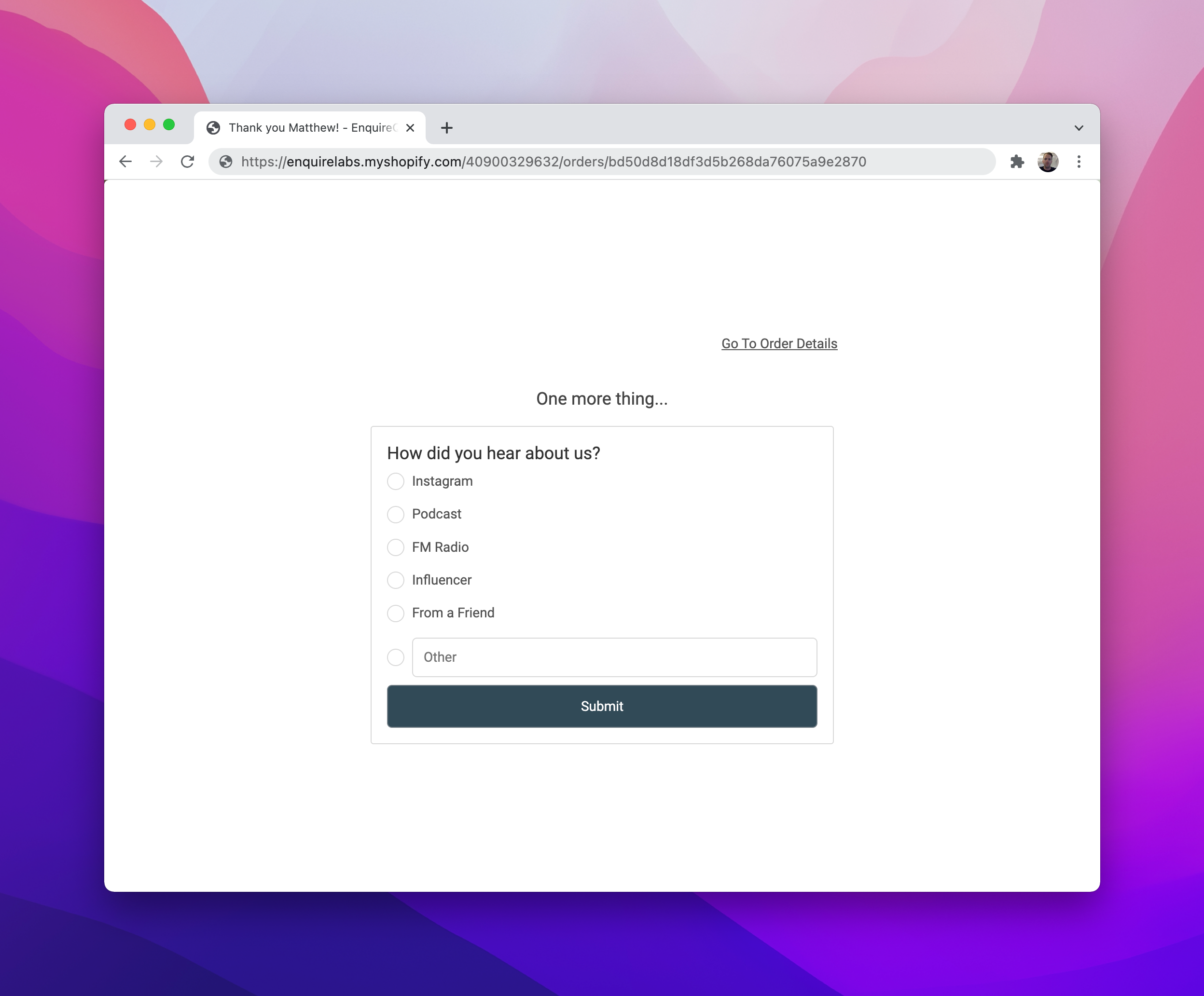
<style>
body.el-no-scroll {
height: 100%;
max-height: 100vh;
overflow-y: hidden;
}
.el-no-scroll .enquire__survey {
border: 1px solid #d9d9d9;
border-radius: 3px;
}
.enquire__action.enquire__action--submit {
background-color: #304a57;
color: #fff;
width: 100%;
}
.enquire__radio-control:checked,
.enquire__radio-control:checked:hover {
border-color: #304a57;
color: #304a57;
}
.enquire__other-response:focus {
box-shadow: 0 0 0 1px #304a57;
border-color: #304a57;
}
/* The container element covers the entire page to provide the
full page takeover */
[role='main']
.main__content
.section
.section__content
.content-box[data-el-container],
.main__content .section .section__content .content-box[data-el-container] {
align-items: center; /* DO NOT MODIFY */
background: white; /* Change this to make the background color
of the takeover match your site's theme */
border: none; /* This removes the default border around the page.
if you'd like a border, change this to something
like "4px solid red" */
bottom: 0; /* DO NOT MODIFY */
box-sizing: border-box; /* DO NOT MODIFY */
display: flex; /* DO NOT MODIFY */
flex-direction: column; /* DO NOT MODIFY */
justify-content: center; /* DO NOT MODIFY */
left: 0; /* DO NOT MODIFY */
overflow-y: scroll; /* DO NOT MODIFY */
position: fixed; /* DO NOT MODIFY */
right: 0; /* DO NOT MODIFY */
top: 0; /* DO NOT MODIFY */
visibility: hidden; /* DO NOT MODIFY */
z-index: 2147483647; /* DO NOT MODIFY */
}
/* The close control element provides a way to hide the full page takeover */
.el-close-control {
background: white; /* Change this value to match the "background"
value you set above */
box-sizing: border-box; /* DO NOT MODIFY */
cursor: pointer; /* DO NOT MODIFY */
margin: 0 0 32px; /* Change this to adjust the whitespace above
and below the "close" control */
max-width: 520px; /* Change this value to adjust the maximum
width of the close control. This value
prevents the close control from displaying at
the far edge of the screen on
larger displays */
padding: 8px 16px; /* Change this value to adjust the spacing around
the text in the close control. These default
values ensure the close control can be easily
clicked */
position: sticky; /* DO NOT MODIFY */
right: 0; /* DO NOT MODIFY */
text-align: right; /* Change this value to adjust where the close
control text appears. Valid values are "left",
"center", or "right" */
text-decoration: underline; /* Change this value to "none" if you don't want
an underline under the text. Not recommended */
top: 0; /* DO NOT MODIFY */
width: 100%; /* DO NOT MODIFY */
z-index: 2147483647; /* DO NOT MODIFY */
}
/* If present, styles the heading text above the question */
.el-heading {
padding-bottom: 20px; /* Add spacing between form and heading text */
text-align: center; /* Remove to left align heading text */
color: #454545; /* Change the color of the heading text */
font-size: 18px; /* Change the size of the heading text */
line-height: 16px; /* Change the spacing betwwen the lines of text
in the heading. Only applies when the heading
text wraps to mulitple lines */
max-width: 480px; /* Prevent the heading from spanning the entire
page on large screens */
width: 100%; /* DO NOT MODIFY */
}
/* Controls the width of the container for the question */
[data-el-container] .enquire__survey {
max-width: 480px; /* Change this to adjust the maximum size of
of the question container. Prevents the
question from "stretching" in width on large
screens */
}
</style>
<script>
(function (window, document) {
const config = {
/*
closeButtonText: "value"
Change this value to be the text you want
to appear on the close control
*/
closeButtonText: 'Go To Order Details',
/*
headerText: "value"
If you would like a header on the full page
takeover, enter the text here. If you don't
want header, remove this value
*/
headerText: 'One more thing...',
/*
successDelay: number;
When the question stream is completed, how
long should the success message display,
in seconds, before the full screen takeover
is hidden
*/
successDelay: 1.5,
/*
takeoverDelay: number
Before the question stream is displayed,
how long to delay displaying the takeover,
in seconds
*/
takeoverDelay: 1,
};
/* YOU SHOULD NOT NEED TO MODIFY ANYTHING BELOW THIS LINE UNLESS
YOU KNOW WHAT YOU'RE DOING */
let dismissed = false;
let container;
let closeControl;
let heading;
function disableModal() {
dismissed = true;
if (!container) return;
container.removeAttribute('data-el-container');
closeControl && closeControl.remove();
heading && heading.remove();
document.body.classList.remove('el-no-scroll');
}
window.addEventListener('fairing.questionStream.initialized', function () {
container = document.querySelector('[data-el-container]');
});
window.addEventListener('fairing.question.displayed', function () {
if (!container) return;
setTimeout(function () {
closeControl = document.createElement('div');
closeControl.innerHTML = config.closeButtonText;
closeControl.classList.add('el-close-control');
document.body.classList.add('el-no-scroll');
if (config.headerText) {
heading = document.createElement('div');
heading.innerHTML = config.headerText;
heading.classList.add('el-heading');
}
heading && container.insertAdjacentElement('afterBegin', heading);
container.insertAdjacentElement('afterBegin', closeControl);
container.style.visibility = 'visible';
closeControl.addEventListener('click', disableModal);
}, config.takeoverDelay * 1000);
});
window.addEventListener('fairing.questionStream.completed', () =>
setTimeout(disableModal, config.successDelay * 1000)
);
})(window, document);
</script>
Updated about 1 month ago