WooCommerce
Option A: Basic Setup
This technique is the least technical if your WooCommerce website is a standard, out-of-the-box installation. This will add the Faring post-purchase survey directly beneath the "Order Received" heading on the WooCommerce order confirmation (thank-you) page.
- You will need to be able to run custom PHP code. The easiest way to do this is download a plugin. We will use the free plugin called Code Snippets.
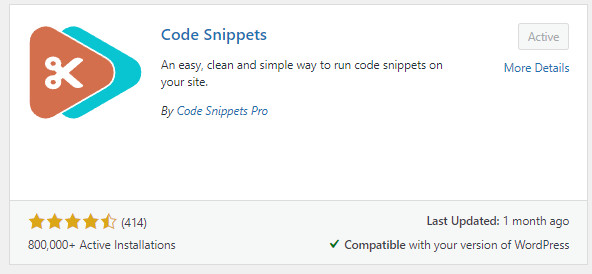
- After installing and activating the plugin, go to the Snippets control panel on the left-hand side of the WordPress admin dashboard and select "Add New.”
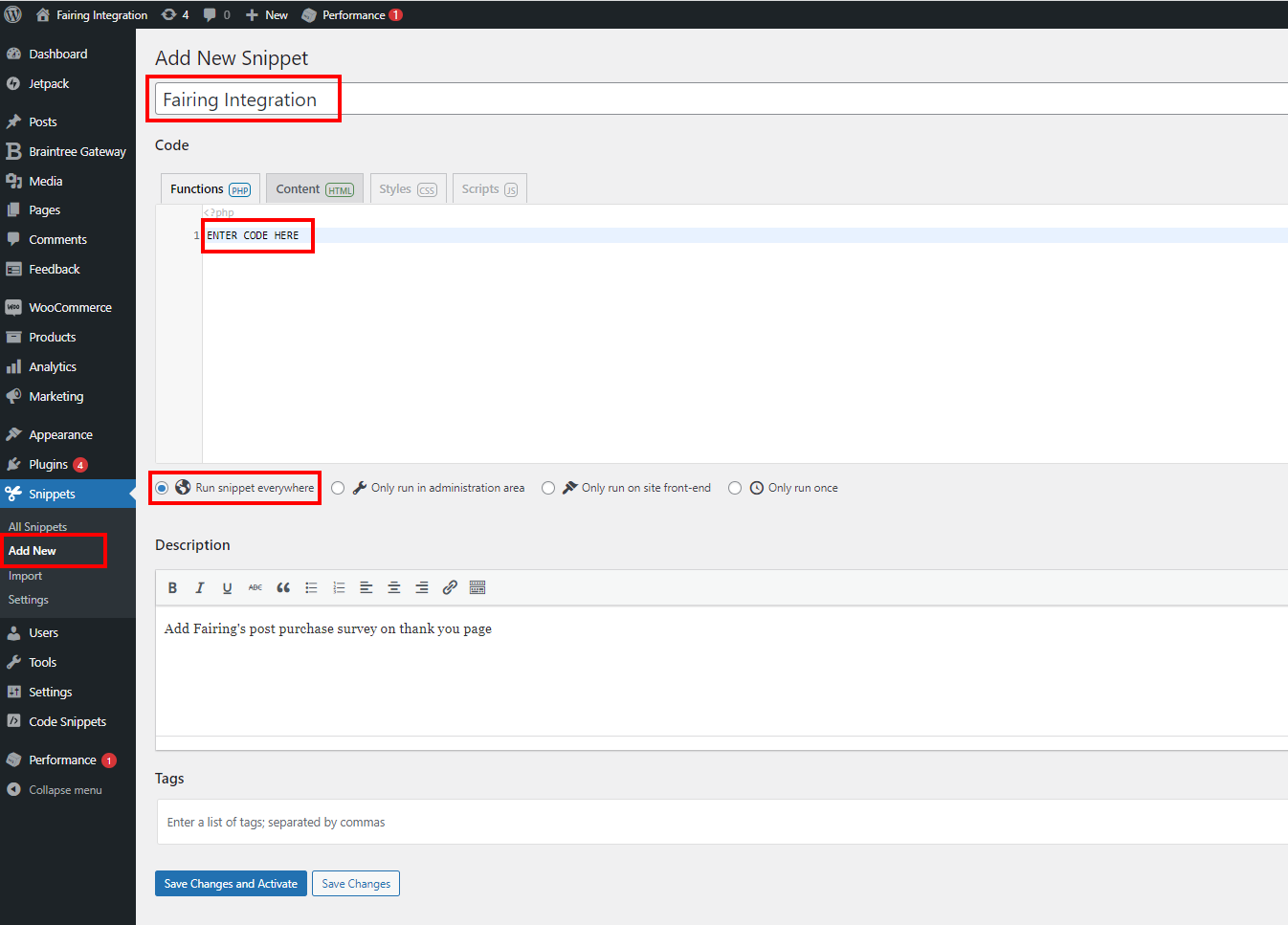
- Give the Code Snippet an appropriate title such as “Fairing Integration”
- Copy the following code in to the function snippet.
NOTE: You must replace<FAIRING PUBLISHABLE API KEY> with your own key provided on your Fairing account page
add_action("woocommerce_thankyou_order_received_text", "thank_you_script_fairing", 20, 2);
if (!function_exists("thank_you_script_fairing")) {
function thank_you_script_fairing($thank_you_title, $order)
{
if ($order instanceof WC_Order) { ?>
<div id="question_stream_target"></div>
<script src="https://app.fairing.co/js/enquire-labs.js"></script>
<script>
const API_KEY = "**<FAIRING PUBLISHABLE API KEY>**";
const TARGET_ELEMENT = document.getElementById("question_stream_target");
// Setup the customer object. We use the customer object to decide which questions to
// ask and to associate responses with a customer.
const customer = {
email: '<?php echo $order->get_billing_email(); ?>', // Included in export data so responses can
// be associated with a customer
id: '<?php echo $order->get_billing_email(); ?>', // Your internal customer ID. Included in export
// data so responses can be associated with a customer
order_count: <?php echo $order->get_user() ? "2" : "1"; ?> // Used to determine if a customer is new or not. If this
// value is 1, the customer is considered new, if it is
// greater than one, the customer is considered returning.
};
// Setup the transaction object. The transaction object allows us to associate order volume
// with responses in reporting, and is included in data exports for more granular analysis.
const transaction = {
country_code: '<?php echo $order->get_shipping_country(); ?>', // REQUIRED The shipping address country code
created_at: '<?php echo date("c", time()); ?>', // REQUIRED The UTC timestamp of when the order was created
currency_code: '<?php echo $order->get_currency(); ?>', // REQUIRED The ISO currency code of the transaction currency
id: '<?php echo $order->get_id(); ?>', // REQUIRED Your internal order ID
locality: '<?php echo $order->get_shipping_city(); ?>', // REQUIRED The shipping address city
number: '<?php echo $order->get_order_number(); ?>', // REQUIRED The transaction number. Useful if your order ID is
// is different from your customer-facing order number
platform: "woocommerce", // REQUIRED The name of the ecommerce platform where the order
// information is stored. For example "magento" or
// "bigcommerce". Any value is valid. It is provided in
// the data export to aid in analysis.
postcode: '<?php echo $order->get_shipping_postcode(); ?>', // REQUIRED The shipping address post code
region: '<?php echo $order->get_shipping_state(); ?>', // REQUIRED The shipping address state or province
source: "website", // REQUIRED The source of the transaction, e.g. "website" or
// "retail store". Any value is valid. Included in
// data export to aid in analysis.
total: '<?php echo $order->get_total(); ?>', // REQUIRED The order total in the local currency
total_usd: '<?php echo $order->get_total(); ?>', // REQUIRED The order total in USD
};
// Wait for the DOM to be ready
window.addEventListener("DOMContentLoaded", function () {
const fairing = Fairing(API_KEY, TARGET_ELEMENT, {
transaction: transaction,
customer: customer,
config: {
integrations: {
ga: false, // Set to true to fire Google Anaylitcs events
gtm: false, // Set to true to fire Google Tag Manger events
rockerbox: false // Set to true to fire Rockerbox events
}
}
});
fairing.nextQuestion();
// To toggle degbug mode. Debug mode will remain enabled until "toggleDebug()" is called
// again, even after page reloads.
// Fairing.toggleDebug()
//
});
</script>
<?php
}
}
}
c. Make sure “Run snippet everywhere” is selected. An optional description can be provided such as “ Add Fairings post purchase survey on thank you page”. This aids in documenting your code/integration should someone else come along in the future. Click “Save Changes and Activate”
- Make a test purchase to verify the integration is working as expected.
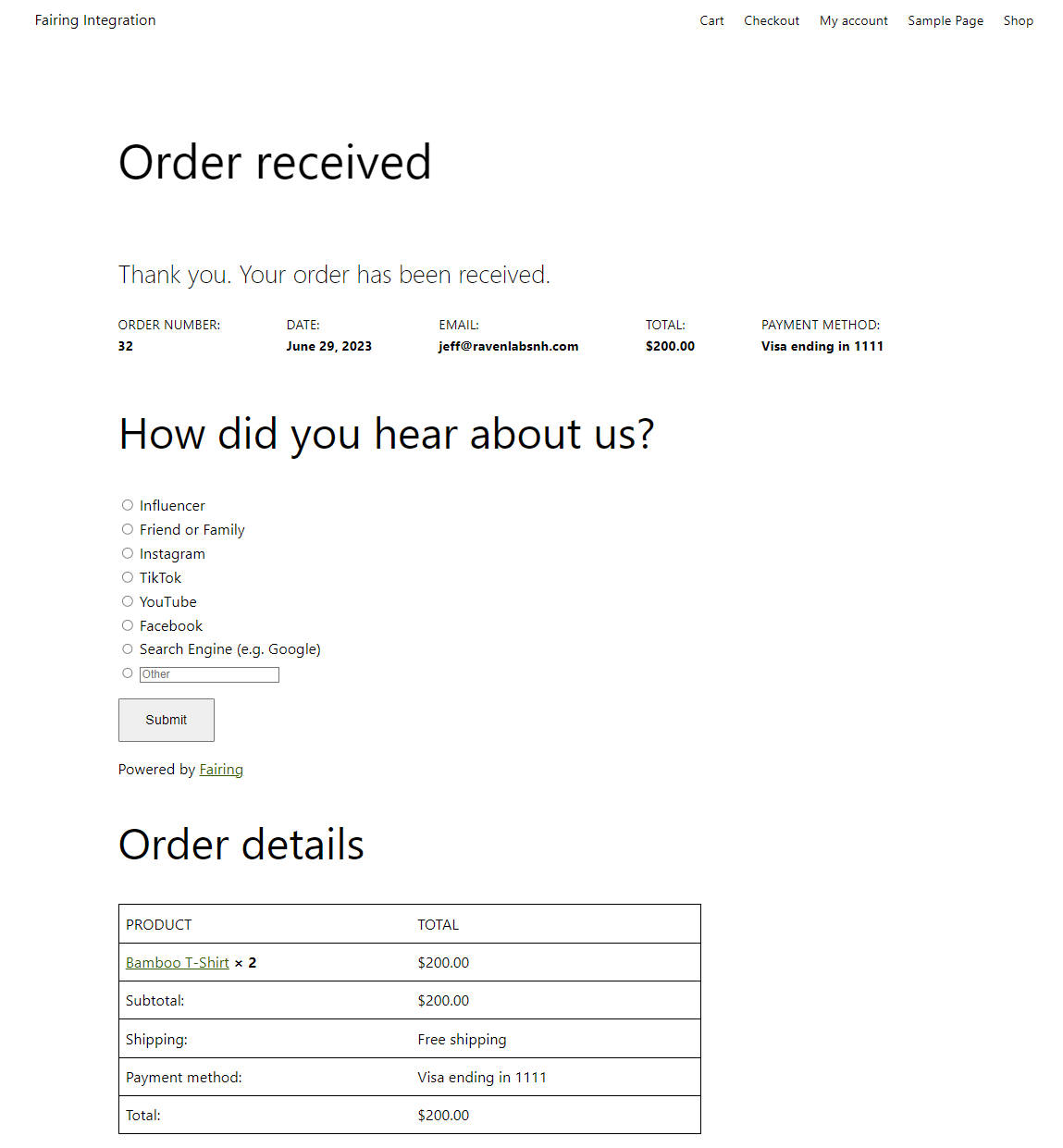
Option B: Intermediate Setup
If your WooCommerce website is more customized, you likely have a custom theme or child theme. In this case, you should add the code excerpt from Option A to your functions.php
file rather than a plugin. This will help reduce conflicts and consolidate custom functionality.
The preceding code snippet operates by utilizing WordPress hook functionality. Here you can learn more about hooks:
Hooks | Plugin Developer Handbook | WordPress Developer Resources
Currently, we are triggering off of the woocommerce_thankyou_order_received_text
action, which, as its name suggests, will place the survey below the "Order Received" text. Additionally, we could also trigger off of woocommerce_thankyou
action which would display the survey right below the order detail block.
Option C: Advanced Setup
If your WooCommerce site requires extensive customization, you may need to modify the template files to include Fairing in order to achieve the desired results. If this is the path you require and you do not have a web developer, we advise you to consult one. In this example, we will provide a basic implementation on how to incorporate the fairing survey in to a template file. The post-purchase survey will still appear on the WooCommerce Thank You page, but in a different location.
- In your custom or child theme, copy over the WooCommerce thank you page template
wp-content/plugins/woocommerce/templates/checkout/thankyou.php
toyourtheme/woocommerce/checkout/thankyou.php.
- In the copied over template file, we can add the Fairing script and html elements. Here, we will place the survey right above the “Order Details” Heading. Below is the default thank you template file
<div class="woocommerce-order">
<?php
if ( $order ) :
do_action( 'woocommerce_before_thankyou', $order->get_id() );
?>
<?php if ( $order->has_status( 'failed' ) ) : ?>
<p class="woocommerce-notice woocommerce-notice--error woocommerce-thankyou-order-failed"><?php esc_html_e( 'Unfortunately your order cannot be processed as the originating bank/merchant has declined your transaction. Please attempt your purchase again.', 'woocommerce' ); ?></p>
<p class="woocommerce-notice woocommerce-notice--error woocommerce-thankyou-order-failed-actions">
<a href="<?php echo esc_url( $order->get_checkout_payment_url() ); ?>" class="button pay"><?php esc_html_e( 'Pay', 'woocommerce' ); ?></a>
<?php if ( is_user_logged_in() ) : ?>
<a href="<?php echo esc_url( wc_get_page_permalink( 'myaccount' ) ); ?>" class="button pay"><?php esc_html_e( 'My account', 'woocommerce' ); ?></a>
<?php endif; ?>
</p>
<?php else : ?>
<p class="woocommerce-notice woocommerce-notice--success woocommerce-thankyou-order-received"><?php echo apply_filters( 'woocommerce_thankyou_order_received_text', esc_html__( 'Thank you. Your order has been received.', 'woocommerce' ), $order ); // phpcs:ignore WordPress.Security.EscapeOutput.OutputNotEscaped ?></p>
<ul class="woocommerce-order-overview woocommerce-thankyou-order-details order_details">
<li class="woocommerce-order-overview__order order">
<?php esc_html_e( 'Order number:', 'woocommerce' ); ?>
<strong><?php echo $order->get_order_number(); // phpcs:ignore WordPress.Security.EscapeOutput.OutputNotEscaped ?></strong>
</li>
<li class="woocommerce-order-overview__date date">
<?php esc_html_e( 'Date:', 'woocommerce' ); ?>
<strong><?php echo wc_format_datetime( $order->get_date_created() ); // phpcs:ignore WordPress.Security.EscapeOutput.OutputNotEscaped ?></strong>
</li>
<?php if ( is_user_logged_in() && $order->get_user_id() === get_current_user_id() && $order->get_billing_email() ) : ?>
<li class="woocommerce-order-overview__email email">
<?php esc_html_e( 'Email:', 'woocommerce' ); ?>
<strong><?php echo $order->get_billing_email(); // phpcs:ignore WordPress.Security.EscapeOutput.OutputNotEscaped ?></strong>
</li>
<?php endif; ?>
<li class="woocommerce-order-overview__total total">
<?php esc_html_e( 'Total:', 'woocommerce' ); ?>
<strong><?php echo $order->get_formatted_order_total(); // phpcs:ignore WordPress.Security.EscapeOutput.OutputNotEscaped ?></strong>
</li>
<?php if ( $order->get_payment_method_title() ) : ?>
<li class="woocommerce-order-overview__payment-method method">
<?php esc_html_e( 'Payment method:', 'woocommerce' ); ?>
<strong><?php echo wp_kses_post( $order->get_payment_method_title() ); ?></strong>
</li>
<?php endif; ?>
</ul>
<?php endif; ?>
\<ADD FAIRING CODE HERE>
<?php do_action( 'woocommerce_thankyou_' . $order->get_payment_method(), $order->get_id() ); ?>
<?php do_action( 'woocommerce_thankyou', $order->get_id() ); ?>
<?php else : ?>
<p class="woocommerce-notice woocommerce-notice--success woocommerce-thankyou-order-received"><?php echo apply_filters( 'woocommerce_thankyou_order_received_text', esc_html__( 'Thank you. Your order has been received.', 'woocommerce' ), null ); // phpcs:ignore WordPress.Security.EscapeOutput.OutputNotEscaped ?></p>
<?php endif; ?>
</div>
- Add the following code to the template (where <ADD FAIRING CODE HERE> is) and don't forget to replace<FAIRING PUBLISHABLE API KEY> with your own key
<div id="question_stream_target"></div>
<script src="https://app.fairing.co/js/enquire-labs.js"></script
<script>
const API_KEY = "<FAIRING PUBLISHABLE API KEY>";
const TARGET_ELEMENT = document.getElementById("question_stream_target");
// Setup the customer object. We use the customer object to decide which questions to
// ask and to associate responses with a customer.
const customer = {
email: '<?php echo $order->get_billing_email(); ?>', // Included in export data so responses can
// be associated with a customer
id: '<?php echo $order->get_billing_email(); ?>', // Your internal customer ID. Included in export
// data so responses can be associated with a customer
order_count: <?php echo $order->get_user() ? "2" : "1"; ?> // Used to determine if a customer is new or not. If this
// value is 1, the customer is considered new, if it is
// greater than one, the customer is considered returning.
};
// Setup the transaction object. The transaction object allows us to associate order volume
// with responses in reporting, and is included in data exports for more granular analysis.
const transaction = {
country_code: '<?php echo $order->get_shipping_country(); ?>', // REQUIRED The shipping address country code
created_at: '<?php echo date("c", time()); ?>', // REQUIRED The UTC timestamp of when the order was created
currency_code: '<?php echo $order->get_currency(); ?>', // REQUIRED The ISO currency code of the transaction currency
id: '<?php echo $order->get_id(); ?>', // REQUIRED Your internal order ID
locality: '<?php echo $order->get_shipping_city(); ?>', // REQUIRED The shipping address city
number: '<?php echo $order->get_order_number(); ?>', // REQUIRED The transaction number. Useful if your order ID is
// is different from your customer-facing order number
platform: "woocommerce", // REQUIRED The name of the ecommerce platform where the order
// information is stored. For example "magento" or
// "bigcommerce". Any value is valid. It is provided in
// the data export to aid in analysis.
postcode: '<?php echo $order->get_shipping_postcode(); ?>', // REQUIRED The shipping address post code
region: '<?php echo $order->get_shipping_state(); ?>', // REQUIRED The shipping address state or province
source: "website", // REQUIRED The source of the transaction, e.g. "website" or
// "retail store". Any value is valid. Included in
// data export to aid in analysis.
total: '<?php echo $order->get_total(); ?>', // REQUIRED The order total in the local currency
total_usd: '<?php echo $order->get_total(); ?>', // REQUIRED The order total in USD
};
// Wait for the DOM to be ready
window.addEventListener("DOMContentLoaded", function () {
const fairing = Fairing(API_KEY, TARGET_ELEMENT, {
transaction: transaction,
customer: customer,
config: {
integrations: {
ga: false, // Set to true to fire Google Anaylitcs events
gtm: false, // Set to true to fire Google Tag Manger events
rockerbox: false // Set to true to fire Rockerbox events
}
}
});
fairing.nextQuestion();
// To toggle degbug mode. Debug mode will remain enabled until "toggleDebug()" is called
// again, even after page reloads.
// Fairing.toggleDebug()
//
});
</script>
- Lets save these files and test it out.
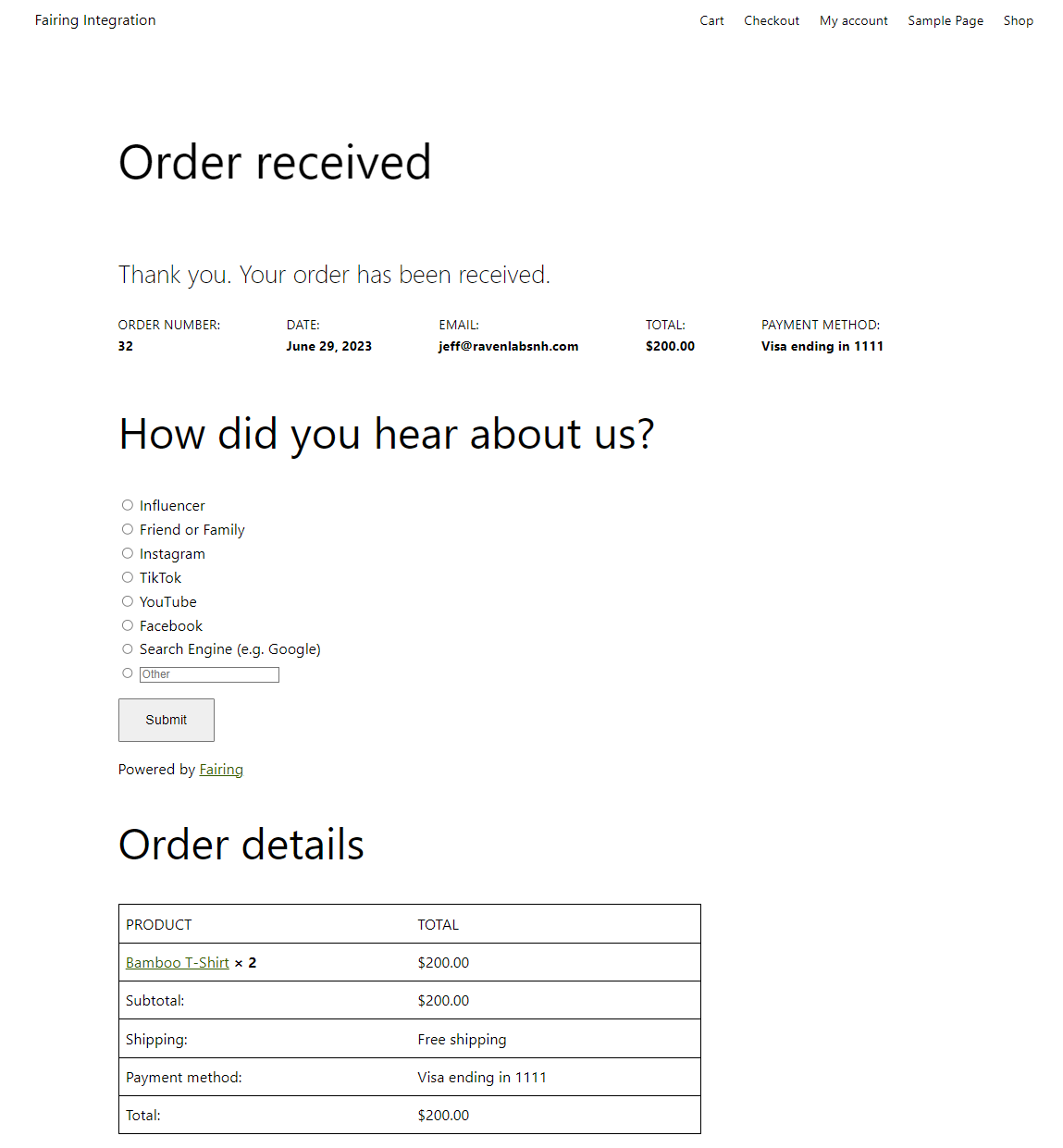
Updated about 1 month ago