Custom Snippet
Integrating Fairing onto any site or checkout.
Not on Shopify? Our custom snippet allows you to integrate Fairing anywhere.
Instructions
Add the script tag
For best results, add the script tag to the head of your document so the script is loaded before the DOM content has finished loading.
<script src="https://app.fairing.co/js/fairing.js">
</script>
Setup DOM element
The script needs a target DOM element to insert the question form into. The DOM element can be included in the page where you would like the questions to appear, or it can be generated programmatically and inserted. The DOM element will be passed as the second argument to the Fairing constructor.
<html>
<head>
...
</head>
<body>
...
<div id="question_stream_target"></div>
...
</body>
</html>
Add script and initialize SDK
Just before the closing body tag, add the below script to initialize the SDK.
Publishable Key 🔑
Your Publishable Key is located under the Account tab in your Fairing admin.
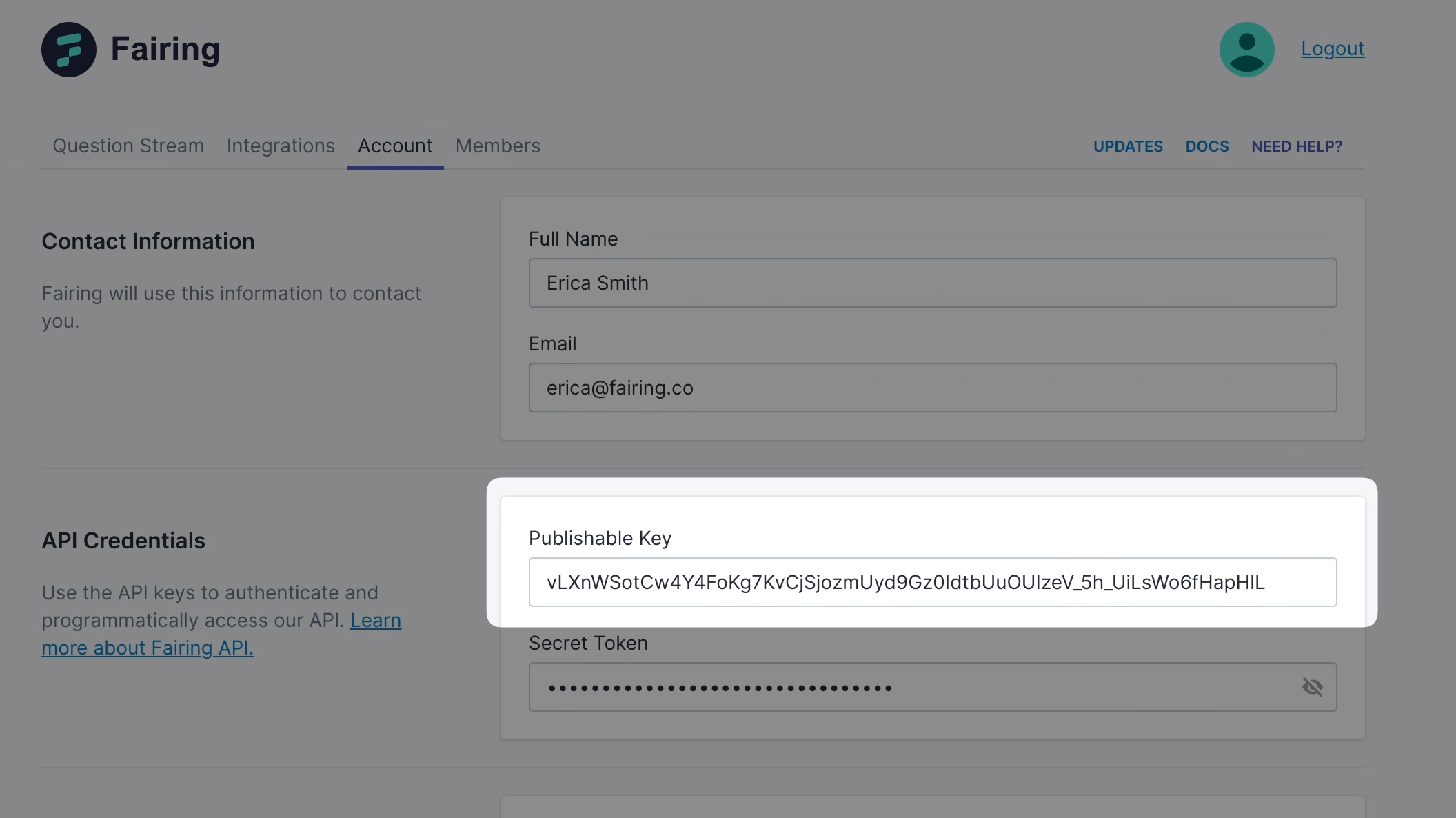
<script>
const API_KEY = "YOUR_PUBLISHABLE_KEY"; // API key (Publishable API key located on your Account page)
const TARGET_ELEMENT = document.getElementById("question_stream_target");
// Setup the customer object. We use the customer object to decide which questions to
// ask and to associate responses with a customer.
const customer = {
email: "[email protected]", // REQUIRED Included in export data so responses can
// be associated with a customer
id: "CUSTOMER ID", // REQUIRED Your internal customer ID. Included in export
// data so responses can be associated with a customer
order_count: 1 // REQUIRED Used to determine if a customer is new or not. If this
// value is 1, the customer is considered new, if it is
// greater than one, the customer is considered returning.
};
// Setup the transaction object. The transaction object allows us to associate order volume
// with responses in reporting, and is included in data exports for more granular analysis.
const transaction = {
country_code: "US", // REQUIRED The shipping address country code
coupon_amount: 10.00, // If a discount was applied, the amount of the discount
coupon_code: "SAVE10", // If a discount was applied, the discount code
coupon_type: "fixed", // This property only accepts "fixed" or "percentage"
created_at: "2021-07-01T10:00:00.00Z", // REQUIRED The UTC timestamp of when the order was created
currency_code: "USD", // REQUIRED The ISO currency code of the transaction currency
id: "1001", // REQUIRED Your internal order ID
landing_page_path: "/products", // The landing page for this transaction
locality: "New York", // REQUIRED The shipping address city
number: "T1001", // REQUIRED The transaction number. Useful if your order ID is
// is different from your customer-facing order number
platform: "ecommerce platform", // REQUIRED The name of the ecommerce platform where the order
// information is stored. For example "magento" or
// "bigcommerce". Any value is valid. It is provided in
// the data export to aid in analysis.
postcode: "10003", // REQUIRED The shipping address post code
referring_url: "https://google.com", // The referring site for the transaction
region: "NY", // REQUIRED The shipping address state or province
source: "website", // REQUIRED The source of the transaction, e.g. "website" or
// "retail store". Any value is valid. Included in
// data export to aid in analysis.
total: 100.00, // REQUIRED The order total in the local currency
utm_campaign: "summer fun", // UTM campaign
utm_content: "splashing waves", // UTM content
utm_medium: "digital", // UTM medium
utm_source: "paid search", // UTM source
utm_term: "summer wine" // UTM term
};
// Wait for the DOM to be ready
window.addEventListener("DOMContentLoaded", function() {
const fairing = Fairing(API_KEY, TARGET_ELEMENT, {
transaction: transaction,
customer: customer,
config: {
integrations: {
ga: false, // Set to true to fire Google Analytics events
gtm: false, // Set to true to fire Google Tag Manager events
rockerbox: false // Set to true to fire Rockerbox events
}
}
});
fairing.nextQuestion();
// To toggle debug mode. Debug mode will remain enabled until "toggleDebug()" is called
// again, even after page reloads.
// Fairing.toggleDebug()
});
</script>
The Transaction Object
- country_code string required
-
The ISO 3166 Alpha-2 country code of the shipping address.
exampleUS
- coupon_amount number
-
If a discount was applied, the amount of the discount in local currency.
example12.99
- coupon_code string
-
If a discount was applied, the discount code.
exampleSALE20
- coupon_type string
-
The type of discount. Must be either
fixed
orpercentage
. - created_at string required
-
The ISO 8601 UTC timestamp when the order was created.
example2023‐07‐26T17:28:18Z
- currency_code string required
-
The ISO 4217 currency code of the transaction.
exampleUSD
- id string required
-
Your platform's internal order ID.
exampleB67Y45389-T76547
- landing_page_path string required
-
The landing page that led to this transaction.
example/shop/fall-sale
- locality string required
-
The shipping address city.
exampleNew York
- number string required
-
The transaction number. Useful if your order ID is is different from your customer-facing order number.
exampleINV10010
- platform string required
-
The name of the ecommerce platform where the order information is stored. For example
magento
orbigcommerce
. Any value is valid. It is provided in the data export to aid in analysis.examplemegento
- postcode string required
-
The shipping address post code.
exampleBN4365
- referring_url string
-
The referring site that led to this transaction.
examplegoogle.com?q=sports
- region string required
-
The 2 letter alpha code for the state or province.
exampleAL
- source string required
-
The source of the transaction, e.g.
website
orretail store
. Any value is valid. Included in data export to aid in analysis.examplewebsite
- total number required
-
The transaction total in the settlement currency.
example34.75
- utm_campaign string
-
The UTM campaign for this transaction.
examplejourney-welcome
- utm_content string
-
The UTM content for this transaction.
exampleproduct-30
- utm_medium string
-
The UTM medium for this transaction.
examplepaid-social
- utm_source string
-
The UTM source for this transaction.
examplegoogle
- utm_term string
-
The UTM term for this transaction.
examplenewsletter
Updated about 1 month ago